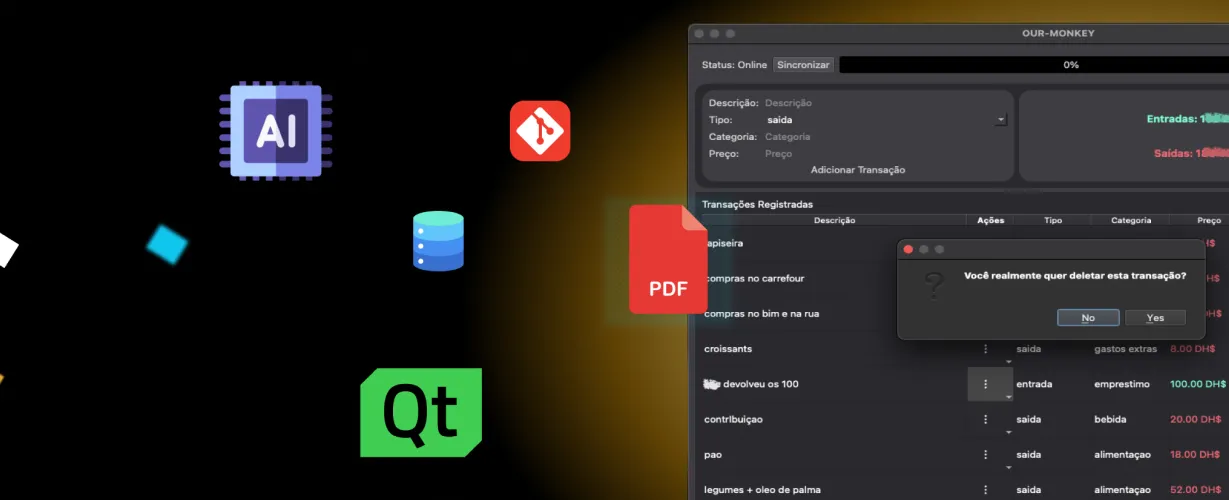
Integrated finance control system
I’m excited to finally share a project I’ve been working on in my spare time: OurMoney, a personal finance tracking app designed for simplicity, efficiency, and offline functionality. Let me tell you about it!
The Problem & My Solution:
Like many, I struggled to find a personal finance app that truly fit my needs. Spreadsheets felt cumbersome, and existing apps were often bloated with unnecessary features or lacked the flexibility I needed. I wanted a solution that was simple to use, accessible on both my computer and phone, and capable of working offline when an internet connection wasn’t available. This led me to create OurMoney.
To meet these requirements, I architected OurMoney as a system of synchronized apps: a desktop application for offline and local use, and a web application for online access.
OurMoney comprises three main components that work together:
- A Desktop App: a GUI app where I can save all expenses and income, whether I’m online or offline.
- A Web App: where I save all expenses and income when I’m online in any other device.
- A Server API: To connect the apps and synchronize data.
Desktop App
desktop GUI built with pyqt
The Desktop App is a GUI built with PyQt and uses a local SQLite database for data storage. It allows the user to add, edit, delete, and view financial transactions, and generate PDF reports based on their data**. To facilitate data analysis, the app also supports exporting transactions to JSON, CSV, and XLSX formats, as well as importing from JSON.
I’ve used pyinstaller to bundle the app and create-dmg to generate the .dmg file
I also added a service using Quarto to build custom pdf reports on click. The data is processed locally and the PDF is generated by the app. you can see how it looks like in the image below. The PDF report includes a table, charts and provides insights into spending patterns.
PDF report generated by the desktop app
The Desktop App synchronizes data with the server using a status flag on each transaction record. The ‘status’ field indicates whether a transaction is ‘unsynced’ (not yet pushed to the server), ‘synced’ (successfully synchronized), ‘deleted’ (locally deleted), or ‘updated’ (locally modified).
In order to ensure the fluidity in GUI, I used some frontend strategies like infinite scroll and threads(the pyQt threads). For example I added a thread in Desktop app that checks the Server API’s availability by sending a ping-pong request periodically. If the Server API is reachable, the app pulls new transactions from the server and saves them locally with a ‘synced’ status. It also pushes local transactions with ‘unsynced,’ ‘deleted,’ or ‘updated’ statuses to the server for synchronization. Because importing big JSON to database and also post/get all this data to/from server by request can take too much time, and memory and processing I used streams and bacthes strategy.
The desktop app architecture follows the Model-View-Controller (MVC) pattern. I separated the use cases as services, and use a data transfer object (DTO) strategy to communicate the services with controller and the GUI.
To back up the database, I used a JSON file to store all transactions. The import service is responsible for reading the JSON file and inserting the transactions into the database in batches.
A snippet of the importing JSON service on MVC:
import ijson
from entities.transactions_entity import TransactionEntity
from repositories.transactions_repository import TransactionsRepository
def import_transactions_to_json():
batch_size = 1000
transactions: list[TransactionEntity] = []
model = TransactionsRepository()
with open('myTransactions_20250120.json', 'r') as file:
parser = ijson.items(file, 'item') # Parse the array of transactions
for transaction in parser:
transaction = TransactionEntity(
id=transaction["id"],
description=transaction["description"],
type=transaction["type"],
category=transaction["category"],
price=float(transaction["price"]),
owner=transaction["owner"],
email=transaction["email"],
created_at=transaction["createdAt"],
status=transaction["status"],
)
transactions.append(transaction)
if len(transactions) >= batch_size:
model.insert_many(transactions)
transactions = []
# Insert any remaining transactions
if transactions:
model.insert_many(transactions)
All the code of the desktop app can be found here
Web App
OurMoney web app built with Reactjs
The Web App provides online access to OurMoney, allowing me to manage my finances from any device with a web browser. Built with ReactJS, the application offers a responsive and intuitive user interface for adding, editing, deleting, and viewing transactions. Because it shall be exposed in internet security is a top priority, I found it interesting to add a security layer with user authentication using JWT (JSON Web Tokens). I automated the deployment process using GitHub Actions to ensure continuous integration and delivery(CI/CD) of updates. This streamlines the deployment, ensuring that updates are pushed efficiently. The Web App integrates with the Server API via RESTful API calls to persist and retrieve transaction data. take a look at the web page here, the code can be found here it uses typescript.
Server API
The Server API acts as the central hub for OurMoney, managing data persistence and synchronization between the Desktop and Web Apps. Built with Node.js and Express, the API provides a RESTful interface for accessing and manipulating transaction data. I chose Node.js and Express for their performance and scalability and above all my experience using it. Data is stored in a PostgreSQL database accessed through Prisma, an ORM that simplifies database interactions and provides type safety. The database schema mirrors the SQLite database used by the Desktop App, ensuring seamless data synchronization. To simplify deployment and ensure consistent environments, the API is containerized using Docker and deployed on Render. Docker Compose is used to manage dependencies locally. I chose Render for its ease of use, cost-effectiveness and robust deployment capabilities.
Final Thoughts:
Building OurMoney was a challenging but rewarding experience. I gained valuable experience with fullstack development, particularly in areas like software architecture, design patterns, data synchronization and security. On the flip side, working on data analysis pushed me to revisit and refresh a bunch of statistics concepts, but was fun. I am proud of achieving a fully functional, cross-platform personal finance application that meets my specific needs. In the future, I plan to add support for automated transaction importing and more advanced reporting features. I welcome any feedback on the project! give me a ⭐ if you like it in my github repo.